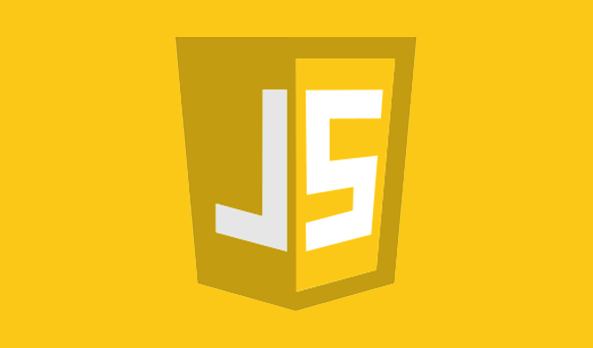
【JavaScript】要素を操作するためのメソッドについて
JavaScriptには、要素を操作するためのさまざまなメソッドがあります。よく使うものをいくつか紹介します。
よく使われるメソッド
document.getElementById
指定されたIDを持つ要素を取得します。
- var element = document.getElementById('myElement');
document.querySelector
CSSセレクタを使用して最初の要素を取得します。
- var element = document.querySelector('.myClass'); // 最初の要素を取得
document.querySelectorAll
CSSセレクタを使用して一致するすべての要素を取得し、NodeListとして返します。
- var elements = document.querySelectorAll('.myClass'); // すべての要素を取得
document.getElementsByClassName
指定されたクラス名を持つすべての要素をHTMLCollectionとして取得します。
- var elements = document.getElementsByClassName('myClass');
document.getElementsByTagName
指定されたタグ名を持つすべての要素をHTMLCollectionとして取得します。
- var elements = document.getElementsByTagName('div');
element.getAttribute
指定された属性の値を取得します。
- var value = element.getAttribute('href');
element.setAttribute
指定された属性に値を設定します。
- element.setAttribute('href', 'https://example.com');
element.classList
クラスを操作するためのメソッド(add, remove, toggle, contains)が含まれています。
- element.classList.add('newClass');
- element.classList.remove('oldClass');
- element.classList.toggle('active');
- var hasClass = element.classList.contains('myClass');
element.innerHTML / element.textContent
要素のHTMLまたはテキストの内容を取得または設定します。
- element.innerHTML = '<p>New Content</p>';
- element.textContent = 'New Content';
element.style
インラインスタイルを設定します。
- element.style.color = 'blue';
- element.style.backgroundColor = 'yellow';
element.innerHTML と element.textContentの使い方の応用例
element.innerHTML
要素のHTMLコンテンツを取得または設定するプロパティです。HTMLタグも含めて扱うことができます。
要素の内容を変更する
- <!DOCTYPE html>
- <html>
- <head>
- <title>innerHTML Example</title>
- </head>
- <body>
- <div id="content">This is the original content.</div>
- <button onclick="changeContent()">Change Content</button>
- <script>
- function changeContent() {
- var element = document.getElementById('content');
- element.innerHTML = '<p>This is the <strong>new</strong> content.</p>';
- }
- </script>
- </body>
- </html>
element.textContent
要素のテキストコンテンツを取得または設定するプロパティです。HTMLタグは無視され、純粋なテキストとして扱われます。
要素のテキストを変更する
- <!DOCTYPE html>
- <html>
- <head>
- <title>textContent Example</title>
- </head>
- <body>
- <div id="content">This is the original content.</div>
- <button onclick="changeText()">Change Text</button>
- <script>
- function changeText() {
- var element = document.getElementById('content');
- element.textContent = 'This is the new text content.';
- }
- </script>
- </body>
- </html>
ユーザーの入力を表示する
- <!DOCTYPE html>
- <html>
- <head>
- <title>User Input Example</title>
- </head>
- <body>
- <input type="text" id="userInput" placeholder="Enter some text">
- <button onclick="displayInput()">Display Input</button>
- <div id="display"></div>
- <script>
- function displayInput() {
- var userInput = document.getElementById('userInput').value;
- var displayElement = document.getElementById('display');
- displayElement.textContent = userInput; // テキストとして表示
- }
- </script>
- </body>
- </html>
テンプレートを使って動的にコンテンツを生成する
- <!DOCTYPE html>
- <html>
- <head>
- <title>Template Example</title>
- </head>
- <body>
- <div id="container"></div>
- <script>
- function addItem(name, description) {
- var container = document.getElementById('container');
- var newItem = `
- <div class="item">
- <h2>${name}</h2>
- <p>${description}</p>
- </div>
- `;
- container.innerHTML += newItem; // HTMLとして追加
- }
- addItem('Item 1', 'This is the description for item 1.');
- addItem('Item 2', 'This is the description for item 2.');
- </script>
- </body>
- </html>
element.textContentのさらに応用した例
aタグがクリックされた時に特定のIDを持つ要素のテキストを変更する
- <!DOCTYPE html>
- <html>
- <head>
- <title>Change Text Example</title>
- </head>
- <body>
- <a href="#" id="changeTextButton">Change Text</a>
- <p id="textElement">This is the original text.</p>
- <script>
- document.getElementById("changeTextButton").addEventListener("click", function(event) {
- event.preventDefault(); // デフォルトの動作(リンク先に飛ぶ)を無効にする
- document.getElementById("textElement").textContent = "This is the new text.";
- });
- </script>
- </body>
- </html>
このコードの説明
1. aタグにIDを付けて、JavaScriptでアクセスできるようにします(例:id=”changeTextButton”)。
2. pタグにもIDを付けて、変更するテキスト要素を指定します(例:id=”textElement”)。
3. JavaScriptのaddEventListenerメソッドを使って、aタグのクリックイベントにリスナーを追加します。
4. クリックイベントが発生した時に、event.preventDefault()を呼び出してデフォルトの動作(リンク先に飛ぶ)を無効にします。
5. document.getElementById(“textElement”).textContentを使って、テキスト要素の内容を新しいテキストに変更します。