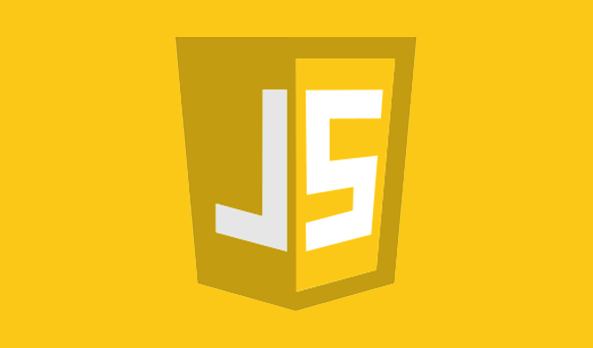
【JAVASCRIPT】オブジェクトを利用したDOM操作サンプルと解説
- const domHelper = {
- element: document.getElementById('sampleElement'),
- setText: function(text) {
- this.element.innerText = text;
- },
- setBackgroundColor: function(color) {
- this.element.style.backgroundColor = color;
- },
- toggleVisibility: function() {
- if (this.element.style.display === 'none') {
- this.element.style.display = 'block';
- } else {
- this.element.style.display = 'none';
- }
- },
- addClass: function(className) {
- this.element.classList.add(className);
- },
- removeClass: function(className) {
- this.element.classList.remove(className);
- }
- };
- // メソッドの使用
- domHelper.setText('DOM WORLD!');
- domHelper.setBackgroundColor('lightblue');
- domHelper.addClass('highlight');
- setTimeout(() => domHelper.toggleVisibility(), 3000);
- setTimeout(() => domHelper.toggleVisibility(), 6000);
- setTimeout(() => domHelper.removeClass('highlight'), 9000);
See the Pen
Untitled by ryotom (@ryotam-smoke)
on CodePen.
目次
オブジェクトの定義と初期化
- const domHelper = {
- element: document.getElementById('sampleElement'),
const domHelper
新しいオブジェクト domHelper を定義しています。const を使っているため、このオブジェクトは再代入されませんが、そのプロパティは変更可能です。
element: document.getElementById(‘sampleElement’)
document.getElementById(‘sampleElement’) により、HTML文書内のIDが sampleElement の要素を取得し、domHelper オブジェクトの element プロパティに代入しています。これにより、他のメソッドでこの要素にアクセスできます。
setText メソッド
- setText: function(text) {
- this.element.innerText = text;
- },
setText: function(text)
text という引数を受け取るメソッド setText を定義しています。
this.element.innerText = text:
this は domHelper オブジェクトを指します。this.element で取得した要素の innerText プロパティを引数 text の値に設定します。これにより、要素の表示テキストが変更されます。
setBackgroundColor メソッド
- setBackgroundColor: function(color) {
- this.element.style.backgroundColor = color;
- },
setBackgroundColor: function(color)
color という引数を受け取るメソッド setBackgroundColor を定義しています。
this.element.style.backgroundColor = color
this.element で取得した要素の style.backgroundColor プロパティを引数 color の値に設定します。これにより、要素の背景色が変更されます。
toggleVisibility メソッド
- toggleVisibility: function() {
- if (this.element.style.display === 'none') {
- this.element.style.display = 'block';
- } else {
- this.element.style.display = 'none';
- }
- },
toggleVisibility: function()
引数を取らないメソッド toggleVisibility を定義しています。
if (this.element.style.display === ‘none’)
this.element.style.display が ‘none’ であれば、要素は非表示状態です。
this.element.style.display = ‘block’
要素を表示状態に変更します。
else { this.element.style.display = ‘none’ }
それ以外の場合、要素を非表示状態に変更します。
このメソッドは、要素の表示/非表示状態をトグル(切り替え)します。
addClass メソッド
- addClass: function(className) {
- this.element.classList.add(className);
- },
addClass: function(className)
className という引数を受け取るメソッド addClass を定義しています。
this.element.classList.add(className)
this.element で取得した要素の classList プロパティに対して add メソッドを呼び出し、引数 className のクラスを追加します。これにより、要素に新しいCSSクラスが追加されます。
removeClass メソッド
- removeClass: function(className) {
- this.element.classList.remove(className);
- }
removeClass: function(className)
className という引数を受け取るメソッド removeClass を定義しています。
this.element.classList.remove(className)
this.element で取得した要素の classList プロパティに対して remove メソッドを呼び出し、引数 className のクラスを削除します。これにより、要素から指定されたCSSクラスが削除されます。
テキストの設定
- domHelper.setText('DOM WORLD!');
この行は、IDが sampleElement の要素のテキストを “DOM WORLD!” に設定します。
背景色の設定
- domHelper.setBackgroundColor('lightblue');
この行は、IDが sampleElement の要素の背景色を lightblue に設定します。
CSSクラスの追加
- domHelper.addClass('highlight');
この行は、IDが sampleElement の要素に highlight というCSSクラスを追加します。
要素の表示/非表示をトグル
- setTimeout(() => domHelper.toggleVisibility(), 3000);
この行は、3秒後にIDが sampleElement の要素の表示/非表示を切り替えます。
再度表示/非表示をトグル
- setTimeout(() => domHelper.toggleVisibility(), 6000);
この行は、6秒後に再度IDが sampleElement の要素の表示/非表示を切り替えます。
CSSクラスの削除
- setTimeout(() => domHelper.removeClass('highlight'), 9000);
この行は、9秒後にIDが sampleElement の要素から highlight というCSSクラスを削除します。
このサンプルでは、DOM要素に対してテキストの変更、背景色の設定、CSSクラスの追加/削除、表示/非表示のトグルといった操作をオブジェクトを使って簡潔に行う方法を示しています。