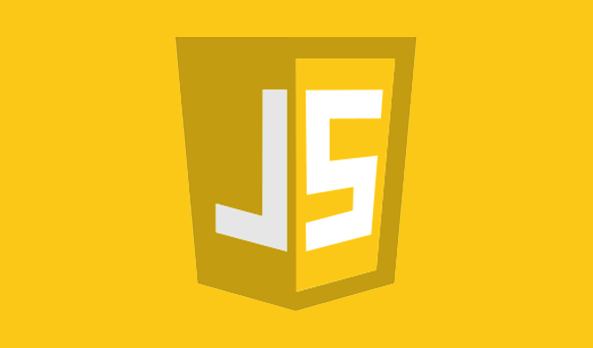
【JAVASCRIPT】10秒ごとに背景色をランダムに変化させるJavascriptの書き方
コード全体の書き方
- <!DOCTYPE html>
- <html lang="ja">
- <head>
- <meta charset="UTF-8">
- <meta name="viewport" content="width=device-width, initial-scale=1.0">
- <title>ランダム背景色変更</title>
- <style>
- body {
- transition: background-color 1s ease;
- }
- </style>
- </head>
- <body>
- <script>
- function getRandomColor() {
- let color;
- do {
- color = `rgb(${Math.floor(Math.random() * 256)}, ${Math.floor(Math.random() * 256)}, ${Math.floor(Math.random() * 256)})`;
- } while (isNearWhiteOrBlack(color));
- return color;
- }
- function isNearWhiteOrBlack(rgb) {
- // RGBを解析
- const rgbValues = rgb.match(/\d+/g).map(Number);
- const [r, g, b] = rgbValues;
- // 色が白に近いか黒に近いかを判断する
- const distanceToWhite = Math.sqrt((255 - r) ** 2 + (255 - g) ** 2 + (255 - b) ** 2);
- const distanceToBlack = Math.sqrt(r ** 2 + g ** 2 + b ** 2);
- return distanceToWhite < 100 || distanceToBlack < 100; // 距離が100未満の場合は白や黒に近いとみなす
- }
- function changeBackgroundColor() {
- document.body.style.backgroundColor = getRandomColor();
- }
- // 初回即時実行
- changeBackgroundColor();
- // 10秒ごとに背景色を変更
- setInterval(changeBackgroundColor, 10000);
- </script>
- </body>
- </html>
getRandomColor関数がランダムなRGB値を生成し、それが白や黒に近い場合は再生成するようにしています。
白や黒に近いかどうかは、各色の距離を計算して判断しています。
そして、changeBackgroundColor関数が背景色を変更します。setIntervalを使って10秒ごとにこの関数を呼び出します。
See the Pen Untitled by ryotom (@ryotam-smoke) on CodePen.
styleでbodyにtransitionを適用させる
- body {
- transition: background-color 1s ease;
- }
背景色の変更が1秒間かけて滑らかに変化するように指定しています。
JavaScriptコード部分の解説
- function getRandomColor() {
- let color;
- do {
- color = `rgb(${Math.floor(Math.random() * 256)}, ${Math.floor(Math.random() * 256)}, ${Math.floor(Math.random() * 256)})`;
- } while (isNearWhiteOrBlack(color));
- return color;
- }
getRandomColor 関数:
ランダムなRGB値を生成し、それを rgb(r, g, b) 形式の文字列として返す関数です。
do…while ループを使って、生成された色が白または黒に近いかどうかを確認し、近い場合は新しい色を生成し直します。
- function isNearWhiteOrBlack(rgb) {
- // RGBを解析
- const rgbValues = rgb.match(/\d+/g).map(Number);
- const [r, g, b] = rgbValues;
- // 色が白に近いか黒に近いかを判断する
- const distanceToWhite = Math.sqrt((255 - r) ** 2 + (255 - g) ** 2 + (255 - b) ** 2);
- const distanceToBlack = Math.sqrt(r ** 2 + g ** 2 + b ** 2);
- return distanceToWhite < 100 || distanceToBlack < 100; // 距離が100未満の場合は白や黒に近いとみなす
- }
isNearWhiteOrBlack 関数:
rgb(r, g, b) 形式の文字列を受け取り、その色が白または黒に近いかどうかを判断します。
rgb 値を解析し、各成分(r, g, b)を取得します。
色が白に近いか黒に近いかを、ユークリッド距離を使って計算します。
白または黒に近い場合は true を返し、そうでない場合は false を返します。
- function changeBackgroundColor() {
- document.body.style.backgroundColor = getRandomColor();
- }
changeBackgroundColor 関数:
getRandomColor 関数を呼び出してランダムな色を取得し、その色を document.body.style.backgroundColor に設定します。
- // 初回即時実行
- changeBackgroundColor();
初回の背景色変更:
changeBackgroundColor 関数を呼び出して、ページ読み込み時に背景色を即時に変更します。
- // 10秒ごとに背景色を変更
- setInterval(changeBackgroundColor, 10000);
10秒ごとに背景色を変更:
setInterval(changeBackgroundColor, 10000) を使って、10秒ごとに changeBackgroundColor 関数を呼び出し、背景色を変更します。